YES, the pari/gp function called qfsign() does use rational numbers only!!!!! You may use it and not fear!
Got a reply from Bill Allombert himself, on a mailing list
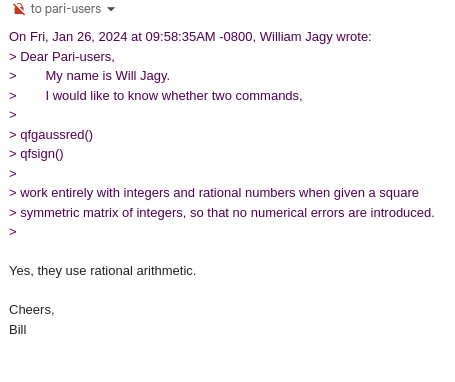
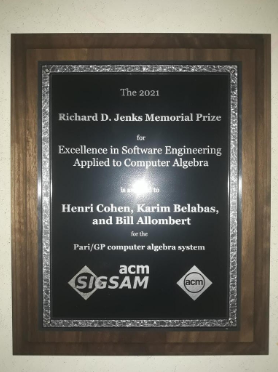
Pari-gp has a version of what I did, naming it after Gauss rather than Lagrange, in the French manner. It is quick, just field operations, so all are rational numbers if you give it integers.
https://fr.wikipedia.org/wiki/R%C3%A9duction_de_Gauss
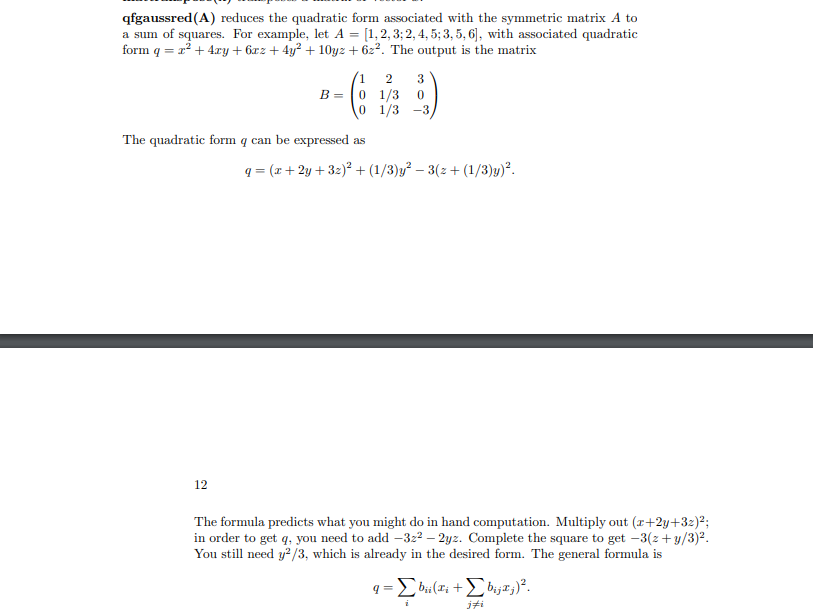
because I can then copy and paste each line and not introduce errors.
Something I have programmed: see comment of Brendan McKay, but as an if and only if... Sylvester's Law of Inertia says that we may decide this if the counts of negative, positive, zero eigenvalues change a little among three symmetric matrices, call them $H, H+I, H-I.$
The hard part was making it give Latex code as output. This is all C++ with GMP; note I wrote my own fractions ... Altogether it is three files, about 200 lines each: myfrac.h /// matrixrational.h /// matrix_congruence.cc
Next given an integer symmetric matrix $H,$ we may solve $P^T H P = D$ diagonal for $P$ nonsingular with rational entries. Once we have $D$ we just count the diagonal elements, how many positive, how many negative, how many zero. The same counts doing the same thing for $H-I$ and $H+I$
$$ H = \left(
\begin{array}{rrrrr}
2 & - 1 & - 1 & - 1 & - 1 \\
- 1 & 2 & 0 & 0 & 0 \\
- 1 & 0 & 2 & 0 & 0 \\
- 1 & 0 & 0 & 2 & 0 \\
- 1 & 0 & 0 & 0 & 2 \\
\end{array}
\right)
$$
$$ D_0 = H $$
$$ E_j^T D_{j-1} E_j = D_j $$
$$ P_{j-1} E_j = P_j $$
$$ E_j^{-1} Q_{j-1} = Q_j $$
$$ P_j Q_j = Q_j P_j = I $$
$$ P_j^T H P_j = D_j $$
$$ Q_j^T D_j Q_j = H $$
$$ H = \left(
\begin{array}{rrrrr}
2 & - 1 & - 1 & - 1 & - 1 \\
- 1 & 2 & 0 & 0 & 0 \\
- 1 & 0 & 2 & 0 & 0 \\
- 1 & 0 & 0 & 2 & 0 \\
- 1 & 0 & 0 & 0 & 2 \\
\end{array}
\right)
$$
$$ \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc $$
$$ P^T H P = D $$
$$\left(
\begin{array}{rrrrr}
1 & 0 & 0 & 0 & 0 \\
\frac{ 1 }{ 2 } & 1 & 0 & 0 & 0 \\
\frac{ 2 }{ 3 } & \frac{ 1 }{ 3 } & 1 & 0 & 0 \\
1 & \frac{ 1 }{ 2 } & \frac{ 1 }{ 2 } & 1 & 0 \\
2 & 1 & 1 & 1 & 1 \\
\end{array}
\right)
\left(
\begin{array}{rrrrr}
2 & - 1 & - 1 & - 1 & - 1 \\
- 1 & 2 & 0 & 0 & 0 \\
- 1 & 0 & 2 & 0 & 0 \\
- 1 & 0 & 0 & 2 & 0 \\
- 1 & 0 & 0 & 0 & 2 \\
\end{array}
\right)
\left(
\begin{array}{rrrrr}
1 & \frac{ 1 }{ 2 } & \frac{ 2 }{ 3 } & 1 & 2 \\
0 & 1 & \frac{ 1 }{ 3 } & \frac{ 1 }{ 2 } & 1 \\
0 & 0 & 1 & \frac{ 1 }{ 2 } & 1 \\
0 & 0 & 0 & 1 & 1 \\
0 & 0 & 0 & 0 & 1 \\
\end{array}
\right)
= \left(
\begin{array}{rrrrr}
2 & 0 & 0 & 0 & 0 \\
0 & \frac{ 3 }{ 2 } & 0 & 0 & 0 \\
0 & 0 & \frac{ 4 }{ 3 } & 0 & 0 \\
0 & 0 & 0 & 1 & 0 \\
0 & 0 & 0 & 0 & 0 \\
\end{array}
\right)
$$
$$ $$
$$ Q^T D Q = H $$
$$\left(
\begin{array}{rrrrr}
1 & 0 & 0 & 0 & 0 \\
- \frac{ 1 }{ 2 } & 1 & 0 & 0 & 0 \\
- \frac{ 1 }{ 2 } & - \frac{ 1 }{ 3 } & 1 & 0 & 0 \\
- \frac{ 1 }{ 2 } & - \frac{ 1 }{ 3 } & - \frac{ 1 }{ 2 } & 1 & 0 \\
- \frac{ 1 }{ 2 } & - \frac{ 1 }{ 3 } & - \frac{ 1 }{ 2 } & - 1 & 1 \\
\end{array}
\right)
\left(
\begin{array}{rrrrr}
2 & 0 & 0 & 0 & 0 \\
0 & \frac{ 3 }{ 2 } & 0 & 0 & 0 \\
0 & 0 & \frac{ 4 }{ 3 } & 0 & 0 \\
0 & 0 & 0 & 1 & 0 \\
0 & 0 & 0 & 0 & 0 \\
\end{array}
\right)
\left(
\begin{array}{rrrrr}
1 & - \frac{ 1 }{ 2 } & - \frac{ 1 }{ 2 } & - \frac{ 1 }{ 2 } & - \frac{ 1 }{ 2 } \\
0 & 1 & - \frac{ 1 }{ 3 } & - \frac{ 1 }{ 3 } & - \frac{ 1 }{ 3 } \\
0 & 0 & 1 & - \frac{ 1 }{ 2 } & - \frac{ 1 }{ 2 } \\
0 & 0 & 0 & 1 & - 1 \\
0 & 0 & 0 & 0 & 1 \\
\end{array}
\right)
= \left(
\begin{array}{rrrrr}
2 & - 1 & - 1 & - 1 & - 1 \\
- 1 & 2 & 0 & 0 & 0 \\
- 1 & 0 & 2 & 0 & 0 \\
- 1 & 0 & 0 & 2 & 0 \\
- 1 & 0 & 0 & 0 & 2 \\
\end{array}
\right)
$$
$$ \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc $$
next $H-I$
$$ P^T H P = D $$
$$\left(
\begin{array}{rrrrr}
1 & 0 & 0 & 0 & 0 \\
2 & 1 & 1 & 0 & 0 \\
0 & - \frac{ 1 }{ 2 } & \frac{ 1 }{ 2 } & 0 & 0 \\
- 1 & - 1 & - 1 & 1 & 0 \\
- \frac{ 1 }{ 2 } & - \frac{ 1 }{ 2 } & - \frac{ 1 }{ 2 } & - \frac{ 1 }{ 2 } & 1 \\
\end{array}
\right)
\left(
\begin{array}{rrrrr}
1 & - 1 & - 1 & - 1 & - 1 \\
- 1 & 1 & 0 & 0 & 0 \\
- 1 & 0 & 1 & 0 & 0 \\
- 1 & 0 & 0 & 1 & 0 \\
- 1 & 0 & 0 & 0 & 1 \\
\end{array}
\right)
\left(
\begin{array}{rrrrr}
1 & 2 & 0 & - 1 & - \frac{ 1 }{ 2 } \\
0 & 1 & - \frac{ 1 }{ 2 } & - 1 & - \frac{ 1 }{ 2 } \\
0 & 1 & \frac{ 1 }{ 2 } & - 1 & - \frac{ 1 }{ 2 } \\
0 & 0 & 0 & 1 & - \frac{ 1 }{ 2 } \\
0 & 0 & 0 & 0 & 1 \\
\end{array}
\right)
= \left(
\begin{array}{rrrrr}
1 & 0 & 0 & 0 & 0 \\
0 & - 2 & 0 & 0 & 0 \\
0 & 0 & \frac{ 1 }{ 2 } & 0 & 0 \\
0 & 0 & 0 & 2 & 0 \\
0 & 0 & 0 & 0 & \frac{ 3 }{ 2 } \\
\end{array}
\right)
$$
$$ $$
$$ Q^T D Q = H $$
$$\left(
\begin{array}{rrrrr}
1 & 0 & 0 & 0 & 0 \\
- 1 & \frac{ 1 }{ 2 } & - 1 & 0 & 0 \\
- 1 & \frac{ 1 }{ 2 } & 1 & 0 & 0 \\
- 1 & 1 & 0 & 1 & 0 \\
- 1 & 1 & 0 & \frac{ 1 }{ 2 } & 1 \\
\end{array}
\right)
\left(
\begin{array}{rrrrr}
1 & 0 & 0 & 0 & 0 \\
0 & - 2 & 0 & 0 & 0 \\
0 & 0 & \frac{ 1 }{ 2 } & 0 & 0 \\
0 & 0 & 0 & 2 & 0 \\
0 & 0 & 0 & 0 & \frac{ 3 }{ 2 } \\
\end{array}
\right)
\left(
\begin{array}{rrrrr}
1 & - 1 & - 1 & - 1 & - 1 \\
0 & \frac{ 1 }{ 2 } & \frac{ 1 }{ 2 } & 1 & 1 \\
0 & - 1 & 1 & 0 & 0 \\
0 & 0 & 0 & 1 & \frac{ 1 }{ 2 } \\
0 & 0 & 0 & 0 & 1 \\
\end{array}
\right)
= \left(
\begin{array}{rrrrr}
1 & - 1 & - 1 & - 1 & - 1 \\
- 1 & 1 & 0 & 0 & 0 \\
- 1 & 0 & 1 & 0 & 0 \\
- 1 & 0 & 0 & 1 & 0 \\
- 1 & 0 & 0 & 0 & 1 \\
\end{array}
\right)
$$
$$ \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc $$
next $H+I$
$$ P^T H P = D $$
$$\left(
\begin{array}{rrrrr}
1 & 0 & 0 & 0 & 0 \\
\frac{ 1 }{ 3 } & 1 & 0 & 0 & 0 \\
\frac{ 3 }{ 8 } & \frac{ 1 }{ 8 } & 1 & 0 & 0 \\
\frac{ 3 }{ 7 } & \frac{ 1 }{ 7 } & \frac{ 1 }{ 7 } & 1 & 0 \\
\frac{ 1 }{ 2 } & \frac{ 1 }{ 6 } & \frac{ 1 }{ 6 } & \frac{ 1 }{ 6 } & 1 \\
\end{array}
\right)
\left(
\begin{array}{rrrrr}
3 & - 1 & - 1 & - 1 & - 1 \\
- 1 & 3 & 0 & 0 & 0 \\
- 1 & 0 & 3 & 0 & 0 \\
- 1 & 0 & 0 & 3 & 0 \\
- 1 & 0 & 0 & 0 & 3 \\
\end{array}
\right)
\left(
\begin{array}{rrrrr}
1 & \frac{ 1 }{ 3 } & \frac{ 3 }{ 8 } & \frac{ 3 }{ 7 } & \frac{ 1 }{ 2 } \\
0 & 1 & \frac{ 1 }{ 8 } & \frac{ 1 }{ 7 } & \frac{ 1 }{ 6 } \\
0 & 0 & 1 & \frac{ 1 }{ 7 } & \frac{ 1 }{ 6 } \\
0 & 0 & 0 & 1 & \frac{ 1 }{ 6 } \\
0 & 0 & 0 & 0 & 1 \\
\end{array}
\right)
= \left(
\begin{array}{rrrrr}
3 & 0 & 0 & 0 & 0 \\
0 & \frac{ 8 }{ 3 } & 0 & 0 & 0 \\
0 & 0 & \frac{ 21 }{ 8 } & 0 & 0 \\
0 & 0 & 0 & \frac{ 18 }{ 7 } & 0 \\
0 & 0 & 0 & 0 & \frac{ 5 }{ 2 } \\
\end{array}
\right)
$$
$$ $$
$$ Q^T D Q = H $$
$$\left(
\begin{array}{rrrrr}
1 & 0 & 0 & 0 & 0 \\
- \frac{ 1 }{ 3 } & 1 & 0 & 0 & 0 \\
- \frac{ 1 }{ 3 } & - \frac{ 1 }{ 8 } & 1 & 0 & 0 \\
- \frac{ 1 }{ 3 } & - \frac{ 1 }{ 8 } & - \frac{ 1 }{ 7 } & 1 & 0 \\
- \frac{ 1 }{ 3 } & - \frac{ 1 }{ 8 } & - \frac{ 1 }{ 7 } & - \frac{ 1 }{ 6 } & 1 \\
\end{array}
\right)
\left(
\begin{array}{rrrrr}
3 & 0 & 0 & 0 & 0 \\
0 & \frac{ 8 }{ 3 } & 0 & 0 & 0 \\
0 & 0 & \frac{ 21 }{ 8 } & 0 & 0 \\
0 & 0 & 0 & \frac{ 18 }{ 7 } & 0 \\
0 & 0 & 0 & 0 & \frac{ 5 }{ 2 } \\
\end{array}
\right)
\left(
\begin{array}{rrrrr}
1 & - \frac{ 1 }{ 3 } & - \frac{ 1 }{ 3 } & - \frac{ 1 }{ 3 } & - \frac{ 1 }{ 3 } \\
0 & 1 & - \frac{ 1 }{ 8 } & - \frac{ 1 }{ 8 } & - \frac{ 1 }{ 8 } \\
0 & 0 & 1 & - \frac{ 1 }{ 7 } & - \frac{ 1 }{ 7 } \\
0 & 0 & 0 & 1 & - \frac{ 1 }{ 6 } \\
0 & 0 & 0 & 0 & 1 \\
\end{array}
\right)
= \left(
\begin{array}{rrrrr}
3 & - 1 & - 1 & - 1 & - 1 \\
- 1 & 3 & 0 & 0 & 0 \\
- 1 & 0 & 3 & 0 & 0 \\
- 1 & 0 & 0 & 3 & 0 \\
- 1 & 0 & 0 & 0 & 3 \\
\end{array}
\right)
$$
$$ \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc \bigcirc $$